2022-02-06 06:02:49 +01:00
|
|
|
import {
|
|
|
|
isArgFormatInvalid,
|
|
|
|
generateRandomSuffix,
|
|
|
|
camelCased,
|
|
|
|
} from './helpers';
|
2020-12-06 18:34:32 +01:00
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
describe('isArgFormatInvalid', () => {
|
|
|
|
test('is false for correct short args', () => {
|
|
|
|
expect(isArgFormatInvalid('-t')).toBe(false);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is true for improperly double-dashed short args', () => {
|
|
|
|
expect(isArgFormatInvalid('--t')).toBe(true);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 22:50:59 +01:00
|
|
|
test('is false for --x and --y (backwards compat, we should have made these short, oh well)', () => {
|
|
|
|
expect(isArgFormatInvalid('--x')).toBe(false);
|
|
|
|
expect(isArgFormatInvalid('--y')).toBe(false);
|
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is false for correct long args', () => {
|
|
|
|
expect(isArgFormatInvalid('--test')).toBe(false);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is true for improperly triple-dashed long args', () => {
|
|
|
|
expect(isArgFormatInvalid('---test')).toBe(true);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is true for improperly single-dashed long args', () => {
|
|
|
|
expect(isArgFormatInvalid('-test')).toBe(true);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is false for correct long args with dashes', () => {
|
|
|
|
expect(isArgFormatInvalid('--test-run')).toBe(false);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
|
2020-12-07 05:19:02 +01:00
|
|
|
test('is false for correct long args with many dashes', () => {
|
|
|
|
expect(isArgFormatInvalid('--test-run-with-many-dashes')).toBe(false);
|
2020-12-06 18:34:32 +01:00
|
|
|
});
|
|
|
|
});
|
Fix injecting multiple css/js files (fix #458) (#1162)
* Add ability to inject multiple css/js files
* API doc: Move misplaced macOS shortcuts doc (PR #1158)
When I added this documentation originally, I guess I placed it in the wrong location.
* README: use quotes in example, to divert users from shell globbing pitfalls
Follow-up of https://github.com/nativefier/nativefier/issues/1159#issuecomment-827184112
* Support opening URLs passed as arg to Nativefied application (fix #405) (PR #1154)
Co-authored-by: Ronan Jouchet <ronan@jouchet.fr>
* macOS: Fix crash when using --tray (fix #527), and invisible icon (fix #942, fix #668) (#1156)
This fixes:
1. A startup crash on macOS when using the `--tray` option; see #527.
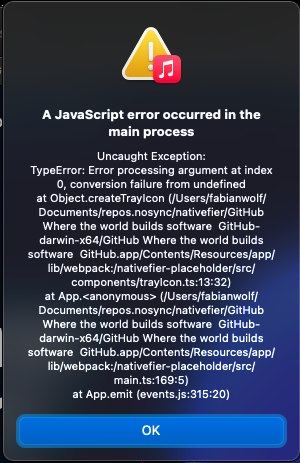
2. Invisible tray icon on macOS; see #942 and #668.
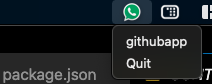
Co-authored-by: Ronan Jouchet <ronan@jouchet.fr>
* API.md / --widevine: document signing apps to make some sites like HBO Max & Udemy work (fix #1147)
* Prompt to confirm when page is attempting to prevent unload (#1163)
Should alleviate part of the issue in #1151
* Add an option to upgrade an existing app (fix #1131) (PR #1138)
This adds a `--upgrade` option to upgrade-in-place an old app, re-using its options it can.
Should help fix #1131
Co-authored-by: Ronan Jouchet <ronan@jouchet.fr>
* Bump to Electron 12.0.5 with Chrome 89.0.4389.128
* Add newly discovered Google internal login page (#1167)
* Fix Widevine by properly listening to widevine-... events, and update docs (fix #1153) (PR #1164)
As documented in #1153, for Widevine support to work properly, we need to listen for the Widevine ready event, and as well for certain sites the app must be signed.
This PR adds the events, and as well adds better documentation on the signing limitation.
This may also help resolve #1147
* Improve suffix creation + tests
* API: clarif in existing doc by the way
* Typo
Co-authored-by: Ronan Jouchet <ronan@jouchet.fr>
Co-authored-by: Ben Curtis <github@nosolutions.com>
Co-authored-by: Fabian Wolf <22625791+fabiwlf@users.noreply.github.com>
2021-04-30 17:04:10 +02:00
|
|
|
|
|
|
|
describe('generateRandomSuffix', () => {
|
|
|
|
test('is not empty', () => {
|
|
|
|
expect(generateRandomSuffix()).not.toBe('');
|
|
|
|
});
|
|
|
|
|
|
|
|
test('is not null', () => {
|
|
|
|
expect(generateRandomSuffix()).not.toBeNull();
|
|
|
|
});
|
|
|
|
|
|
|
|
test('is not undefined', () => {
|
|
|
|
expect(generateRandomSuffix()).toBeDefined();
|
|
|
|
});
|
|
|
|
|
|
|
|
test('is different per call', () => {
|
|
|
|
expect(generateRandomSuffix()).not.toBe(generateRandomSuffix());
|
|
|
|
});
|
|
|
|
|
|
|
|
test('respects the length param', () => {
|
|
|
|
expect(generateRandomSuffix(10).length).toBe(10);
|
|
|
|
});
|
|
|
|
});
|
2022-02-06 06:02:49 +01:00
|
|
|
|
|
|
|
describe('camelCased', () => {
|
|
|
|
test('has no hyphens in camel case', () => {
|
|
|
|
expect(camelCased('file-download')).toEqual(expect.not.stringMatching(/-/));
|
|
|
|
});
|
|
|
|
|
|
|
|
test('returns camel cased string', () => {
|
|
|
|
expect(camelCased('file-download')).toBe('fileDownload');
|
|
|
|
});
|
|
|
|
|
|
|
|
test('has no spaces in camel case', () => {
|
|
|
|
expect(camelCased('--file--download--')).toBe('fileDownload');
|
|
|
|
});
|
|
|
|
|
|
|
|
test('handles multiple hyphens properly', () => {
|
|
|
|
expect(camelCased('file--download--options')).toBe('fileDownloadOptions');
|
|
|
|
});
|
|
|
|
|
|
|
|
test('does not affect non-snake cased strings', () => {
|
|
|
|
expect(camelCased('win32options')).toBe('win32options');
|
|
|
|
});
|
|
|
|
});
|